10 Sep 2020
Get list of referenced files
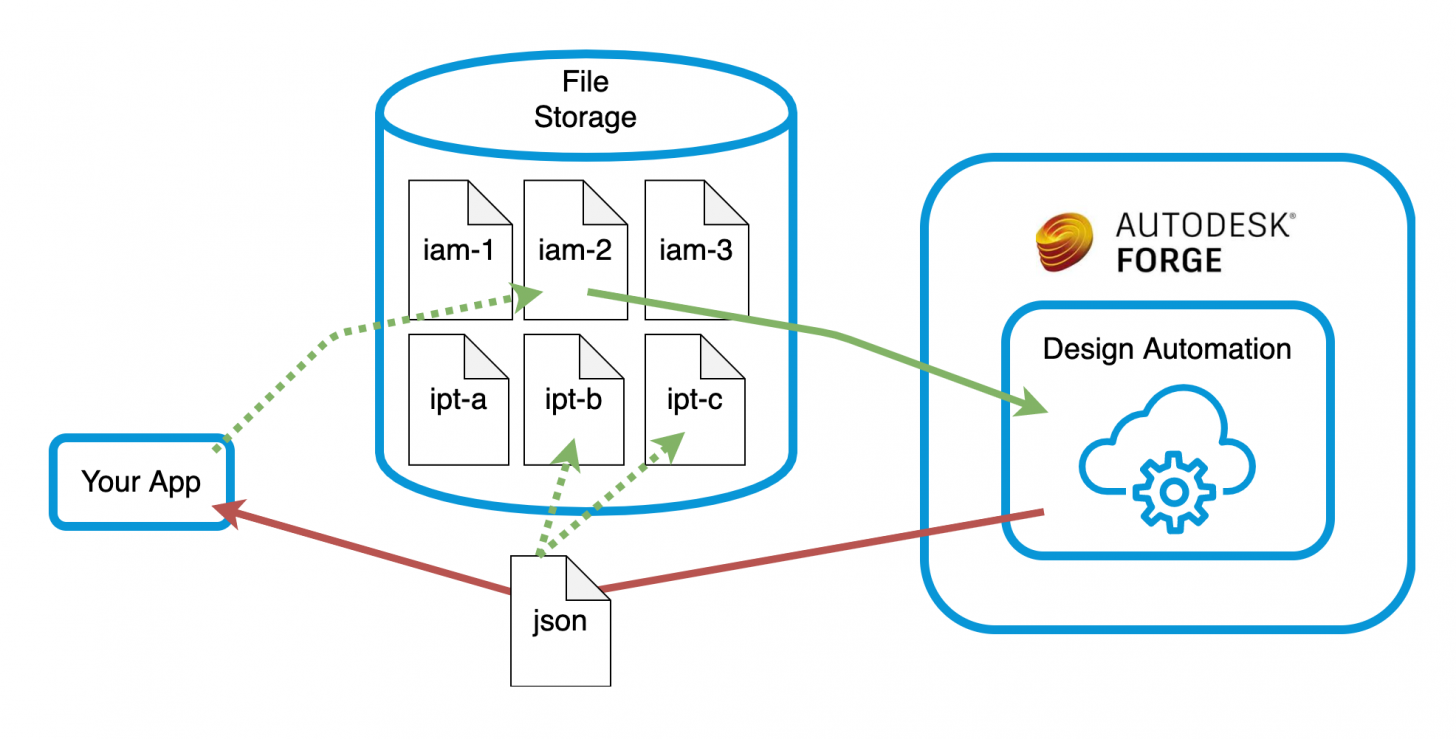
When working with documents, one problem might be not knowing what other documents they reference.
E.g. if you want to translate an Inventor assembly to SVF using the Model Derivative service, then you'll need all the referenced subassemblies and parts it's using. You can either upload all the relevant files inside a zip or upload them separately and set up the references between them - see https://forge.autodesk.com/blog/translate-composite-models-files-references
If you have all the files locally and Inventor is installed then you can simply use the Document.File.AllReferencedFiles property provided by Inventor API to find all the references. You could also use the "Pack and Go" functionality of Inventor to zip up all the necessary files for you.
However, if you try to do this from a web server that does not have all the files locally, and you are dealing with a multi-level assembly then things get a bit more complicated.
Using Design Automation API for Inventor you can write an AppBundle that can list the files directly referenced by the input document. Since none of the referenced documents would be available on the Design Automation server (since we'd only provide the main assembly) therefore Document.File.AllReferencedFiles would be empty, but Document.File.ReferencedFileDescriptors would provide the list of direct references.
Then we'd have to keep repeating the above method for all the subassemblies (and sub-subassemblies, etc) until we gather all the dependencies of the main assembly.
Here are the properties of the Activity we could use:
(note: there are quotation marks " around the $(args[InventorDoc].path) part so that things work even if the file name of the assembly document contains spaces)
{
"commandLine": [
"$(engine.path)\\InventorCoreConsole.exe /al $(appbundles[FileReferencesDA].path) /i \"$(args[InventorDoc].path)\""
],
"parameters": {
"InventorDoc": {
"verb": "get",
"description": "File to process"
},
"OutputJson": {
"verb": "put",
"description": "Json with with file references",
"localName": "references.json"
}
},
"engine": "Autodesk.Inventor+2021",
"appbundles": [
"rGm0mO9jVSsD2yBEDk9MRtXQTwsa61y0.FileReferencesDA+alpha"
],
"description": "Gets list of referenced files"
}
And the relevant part of the AppBundle code to find the direct references of an assembly document:
public void Run(Document doc)
{
try
{
using (new HeartBeat())
{
using (var file = new System.IO.StreamWriter(@"references.json"))
{
var ja = new Newtonsoft.Json.Linq.JArray();
foreach (Inventor.FileDescriptor fd in doc.File.ReferencedFileDescriptors)
{
ja.Add(fd.FullFileName);
}
file.Write(ja.ToString());
}
}
}
catch (Exception e)
{
LogError("Processing failed. " + e.ToString());
}
}
Best to create the above project using our Visual Studio Project Template: https://forge.autodesk.com/blog/design-automation-inventor-vs-template
Here is the finished project: https://github.com/adamenagy/Container/blob/master/FileReferencesDA.zip