7 Apr 2018
3legged token process in Node.js cli
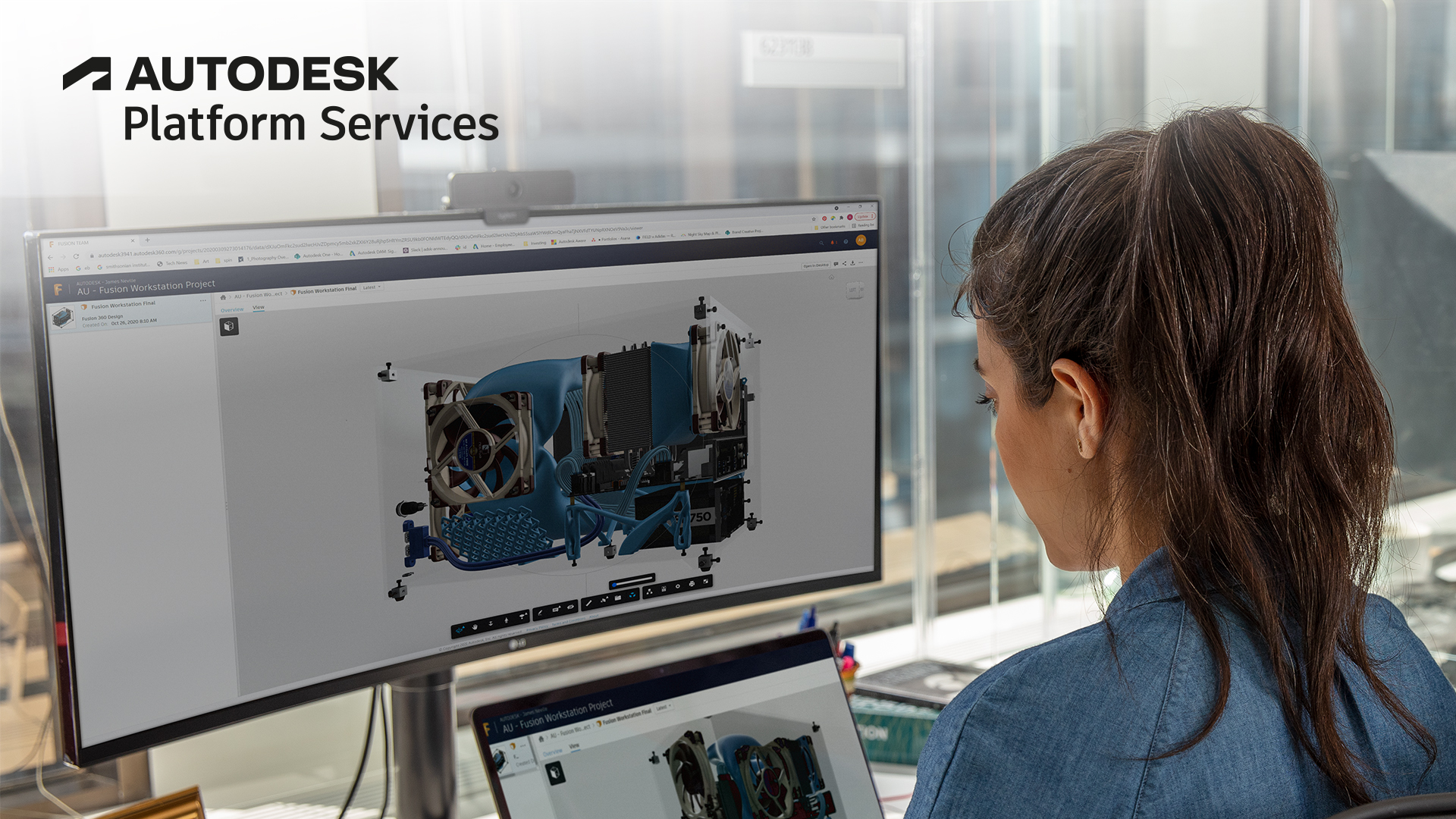
In my recent commitment, I made a Node.js script to test a workflow with 3legged token in Node.js cli. The Node.js SDK of Forge provides a demo on 2legged token, but not yet provided demo of 3legged. While with the hints from .NET SDK of Forge, I produced the similar workflow in Node.js. It is pretty easy: simply start an HTTP listener of the callback endpoint, pop out the webpage for authorization by opn, after the user granted, redirect to callback endpoint to get the token.
The complete test is available at: https://github.com/xiaodongliang/Forge-3legged-Node-Cli.
The below are some core codes. The test also encloses the workflow to refresh the access token in a specific time internal.
1. token process:
//for pop out oAuth log in dialog
var opn = require('opn');
//......
//pop out oAuth log in dialog
function startoAuth()
{
var url =
"https://developer.api.autodesk.com" +
'/authentication/v1/authorize?response_type=code' +
'&client_id=' + config.credentials.client_id +
'&redirect_uri=' + config.callbackURL +
'&scope=' + config.scope.join(" ");
//pop out the dialog of use login and authorization
opn(url, function (err) {
if (err) throw err;
console.log('The user closed the browser');
});
}
//callback endpoint
router.get('/forgeoauth',function(req,res){
//Authorization Code
var code = req.query.code;
var forge3legged = new forgeSDK.AuthClientThreeLegged(
config.credentials.client_id,
config.credentials.client_secret,
config.callbackURL,
config.scope);
forge3legged.getToken(code).then(function (tokenInfo) {
//write token and refresh token to a file
writeTokenFile(tokenInfo);
res.redirect('/')
}).catch(function (err) {
console.log(err);
res.redirect('/')
});
});
module.exports = {
router:router,
startoAuth:startoAuth
};
2. http server:
var express = require('express');
var app = express();
var server = require('http').Server(app);
//get token route
var tokenRoute = require('./token');
app.use('/token', tokenRoute.router);
//set port
app.set('port', process.env.PORT || 1234);
server.listen(app.get('port'), function() {
console.log('Server listening on port '
+ server.address().port);
});
//start oAuth process
tokenRoute.startoAuth();