9 May 2019
Basic point clouds in Forge Viewer
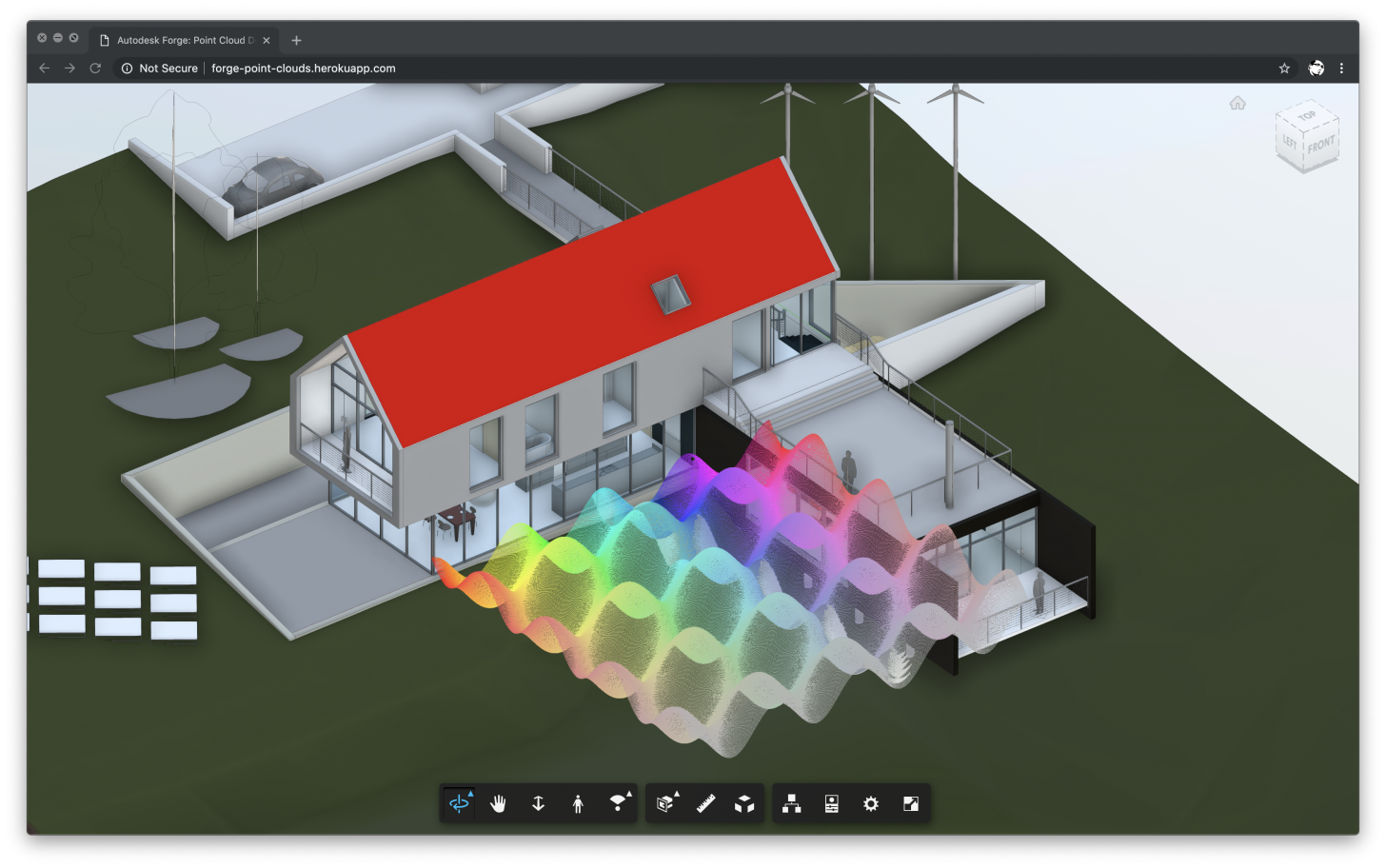
Developers often ask us whether point clouds are supported in Forge Viewer. Unfortunately, the official answer is "no". The focus of Forge Viewer has always been line and mesh geometry, and that is also what the implementation is optimized for. For large scale point cloud rendering on the web, we would suggest 3rd party solutions such as potree or plas.io.
With that said, you can visualize smaller scale point clouds in Forge Viewer through the functionality in the three.js v71 library on which the Viewer is based. The three.js feature in question is the PointCloud class. Here's how you can use it to generate a point cloud object and insert it into the viewer scene:
const GridWidth = 1000;
const GridHeight = 1000;
const PointsCount = GridWidth * GridHeight;
const PointSize = 0.1;
const positions = new Float32Array(PointsCount * 3);
const colors = new Float32Array(PointsCount * 3);
let i = 0;
for (var x = 0; x < GridWidth; x++) {
for (var y = 0; y < GridHeight; y++) {
const u = x / GridWidth, v = y / GridHeight;
positions[3 * i] = u;
positions[3 * i + 1] = v;
positions[3 * i + 2] = 0.0;
colors[3 * i] = u;
colors[3 * i + 1] = v;
colors[3 * i + 2] = 0.0;
i++;
}
}
const geometry = new THREE.BufferGeometry();
geometry.addAttribute('position', new THREE.BufferAttribute(positions, 3));
geometry.addAttribute('color', new THREE.BufferAttribute(colors, 3));
geometry.computeBoundingBox();
geometry.isPoints = true; // This flag is important! It will force Forge Viewer to render the geometry as gl.POINTS
const material = new THREE.PointCloudMaterial({ size: PointSize, vertexColors: THREE.VertexColors });
const points = new THREE.PointCloud(geometry, material);
viewer.impl.createOverlayScene('pointclouds');
viewer.impl.addOverlay('pointclouds', points);
We've prepared a self-contained Forge application sample that uses the PointCloud class to render 1 million colored points within a Revit model. The source code of the sample is available at https://github.com/petrbroz/forge-point-clouds, and a live demo of the same sample is running at https://forge-point-clouds.herokuapp.com.
Note: If you're interested in dynamic point clouds, check out the experimental git branch experiment/streaming. The code implements a simple Websocket server, and the viewer keeps updating parts of its point cloud buffer in predefined time intervals with data from the server.
Browser support
The WebGL support for point clouds has been tested in several combinations of platforms and browsers. In general, point rendering is available in most of the evergreen browsers, however in some cases it seems that the point size is not supported, so keep that in mind.