31 Oct 2018
Upload files to BIM 360 in C#
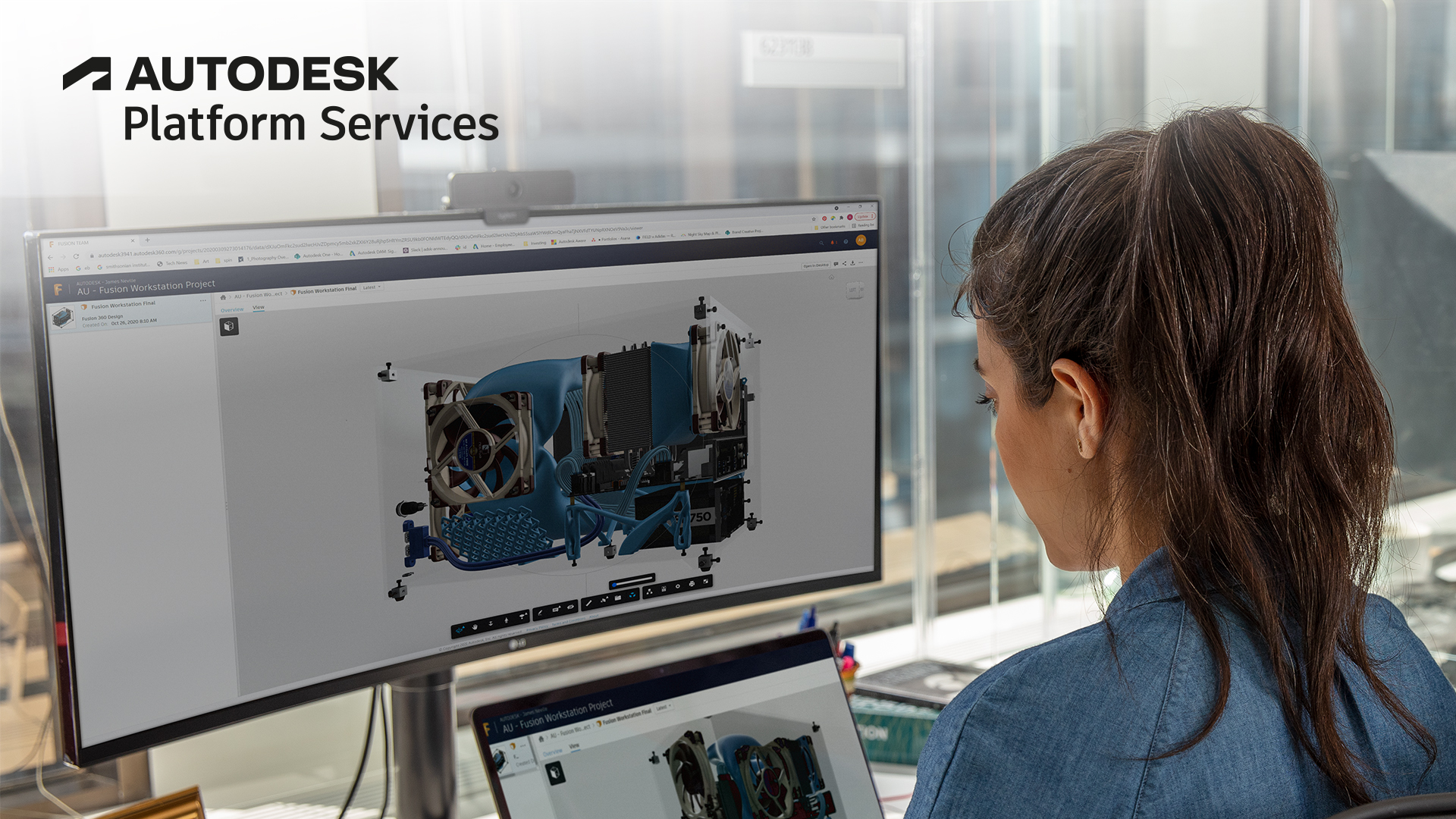
Upload files to Data Management API require a few steps, described at this tutorial:
- Define where to upload the file (hub & project & folder)
- At the project, create a new storage location
- Upload the file to this location
- Create a version (first or new)
This process can be done with any programming language, this post shows the code for .NET.
1. Hubs, Projects & folders
To define the hub/project/folder we can navigate the hierarchy, something as described at this tutorial.
2. Define storage location
For this section we'll need a valid 3-legged token, defined as userAccessToken variable. We'll also need the projectId and itemId. The following code also breaks the storage into an uploadUrl that can be used to put the file.
// get item
ItemsApi itemApi = new ItemsApi();
itemApi.Configuration.AccessToken = userAccessToken;
dynamic item = await itemApi.GetItemAsync(projectId, itemId);
string folderId = item.data.relationships.parent.data.id;
string fileName = item.data.attributes.displayName;
// prepare storage
ProjectsApi projectApi = new ProjectsApi();
projectApi.Configuration.AccessToken = userAccessToken;
StorageRelationshipsTargetData storageRelData = new StorageRelationshipsTargetData(StorageRelationshipsTargetData.TypeEnum.Folders, folderId);
CreateStorageDataRelationshipsTarget storageTarget = new CreateStorageDataRelationshipsTarget(storageRelData);
CreateStorageDataRelationships storageRel = new CreateStorageDataRelationships(storageTarget);
BaseAttributesExtensionObject attributes = new BaseAttributesExtensionObject(string.Empty, string.Empty, new JsonApiLink(string.Empty), null);
CreateStorageDataAttributes storageAtt = new CreateStorageDataAttributes(fileName, attributes);
CreateStorageData storageData = new CreateStorageData(CreateStorageData.TypeEnum.Objects, storageAtt, storageRel);
CreateStorage storage = new CreateStorage(new JsonApiVersionJsonapi(JsonApiVersionJsonapi.VersionEnum._0), storageData);
dynamic storageCreated = await projectApi.PostStorageAsync(projectId, storage);
string[] storageIdParams = ((string)storageCreated.data.id).Split('/');
string[] bucketKeyParams = storageIdParams[storageIdParams.Length - 2].Split(':');
string bucketKey = bucketKeyParams[bucketKeyParams.Length - 1];
string objectName = storageIdParams[storageIdParams.Length - 1];
string uploadUrl = string.Format("https://developer.api.autodesk.com/oss/v2/buckets/{0}/objects/{1}", bucketKey, objectName);
3. Upload the file
This can be done with a simple PUT call or using resumable approach, as described on this other blog post.
4. Create version
This must be done after the file complete the upload. The following code uses the storageId (from step 2), itemId and fileName .
VersionsApi versionsApis = new VersionsApi();
versionsApis.Configuration.AccessToken = credentials.TokenInternal;
CreateVersion newVersionData = new CreateVersion
(
new JsonApiVersionJsonapi(JsonApiVersionJsonapi.VersionEnum._0),
new CreateVersionData
(
CreateVersionData.TypeEnum.Versions,
new CreateStorageDataAttributes
(
fileName,
new BaseAttributesExtensionObject
(
"versions:autodesk.bim360:File",
"1.0",
new JsonApiLink(string.Empty),
null
)
),
new CreateVersionDataRelationships
(
new CreateVersionDataRelationshipsItem
(
new CreateVersionDataRelationshipsItemData
(
CreateVersionDataRelationshipsItemData.TypeEnum.Items,
itemId
)
),
new CreateItemRelationshipsStorage
(
new CreateItemRelationshipsStorageData
(
CreateItemRelationshipsStorageData.TypeEnum.Objects,
storageId
)
)
)
)
);
dynamic newVersion = await versionsApis.PostVersionAsync(projectId, newVersionData);